Node.js 서버 환경이 구축되었으니 이제 간단한 웹서버를 만들어서 동작하는지 확인해보자.
준비사항
nodejs Web Sever 파일을 저장할 디렉토리를 정한다.
편의상 기존 Web 서버의 하위 디렉토리에 두는 것이 여러모로 편할 거 같아서 /usr/local/apache/htdocs/nodejs 디렉토리를 생성했다.
cd /usr/local/apache/htdocs/nodejs
이제 샘플코드를 https://nodejs.org/en/about/ 에서 샘플로 제공한 코드를 EditPlus 에 복사한 다음 코드를 수정했다.
const http = require('http');
// http 모듈에 들어있는 서버 기능을 사용하려면 require()메소드로 http모듈을 불러온다.
const hostname = '127.0.0.1'; // 실제 사용 IP로 변경해야 정상 동작됨
const port = 3000;
// http 객체의 createServer() 메소드를 호출하면 서버 객체가 반환된다.
http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Welcome to Nodejs Server!!!\n');
}).listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
// 이 서버 객체의 listen() 메소드를 호출하면 웹서버가 시작된다.
|
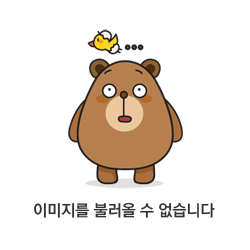
vi webserver.js 파일을 생성한다.
위에 수정한 코드를 붙여넣기하고 :wq 로 저장하고 빠져나온다.
파일을 실행한다.
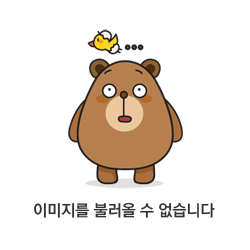
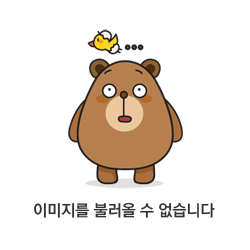
Server running 이라고 화면에 나온다.
이제 Web 브라우저에서 접속해보자.
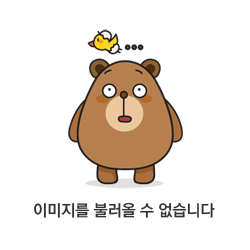
라고 화면에 출력되는 것을 확인할 수 있다.
Node.js 가 잘 동작하는 걸 확인할 수 있다.
백그라운드 실행
#node webserver.js &
프로세스 동작 여부 확인
#ps -ef | grep node
프로세스 죽이기
#kill -p PID번호
#####################################################
express 설치를 해서 Web 서버 만들기
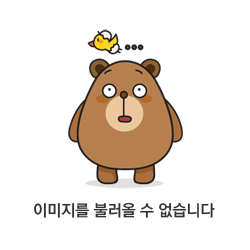
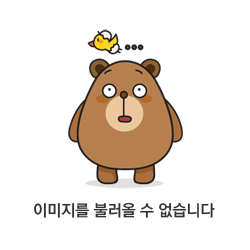
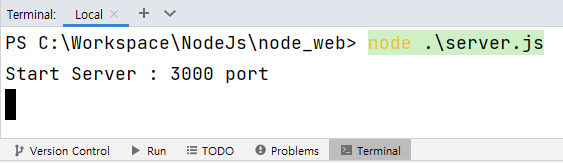
express 모듈은 request 이벤트 리스너를 연결하는데 use() 메소드를 사용한다.
use() 메소드는 여러번 사용할 수 있다.
use() 메소드의 매개변수에는 function(request, response, next) { } 형태의 함수를 입력한다.
use() 메소드의 매개변수에 입력하는 함수를 미들웨어라고 부른다.
요청의 응답을 완료하기 전까지 요청 중간 중간에 여러가지 일을 처리할 수 있다.
// server.js 파일 (Terminal 창에서 아래 두줄 실행한다)
// npm install express --save
// npm install ejs --save
const express = require('express');
const app = express();
const server = app.listen(3000,() => {
console.log('Start Server : 3000 port');
});
app.set('views', __dirname + '/views');
app.set('view engine', 'ejs');
app.engine('html', require('ejs').renderFile);
app.get('/', function (req, res) {
res.render('index.html');
});
app.get('/about', function (req, res) {
res.send('About Page');
});
app.get('/intro', function (req, res) {
res.render('intro.html');
});
|
index.html 파일 → https://www.w3schools.com/ 사이트의 Bootstrap 예제를 가져온 예시 파일이다.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Bootstrap Example</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.1/dist/css/bootstrap.min.css">
<script src="https://cdn.jsdelivr.net/npm/bootstrap@4.6.1/dist/js/bootstrap.bundle.min.js"></script>
</head>
<body>
<div class="container">
<h1>Welcome to My Homepage</h1>
<h2>Basic Table</h2>
<p>The .table class adds basic styling (light padding and horizontal dividers) to a table:</p>
<table class="table">
<thead>
<tr>
<th>Firstname</th>
<th>Lastname</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<tr>
<td>John</td>
<td>Doe</td>
<td>john@example.com</td>
</tr>
<tr>
<td>Mary</td>
<td>Moe</td>
<td>mary@example.com</td>
</tr>
<tr>
<td>July</td>
<td>Dooley</td>
<td>july@example.com</td>
</tr>
</tbody>
</table>
</div>
</body>
</html>
|
'node.js' 카테고리의 다른 글
Node.js 파일 업로드 기능 만들기 (0) | 2018.10.15 |
---|---|
Node.js 서버 chat.js 코드와 Web Chat 클라이언트 코드 (0) | 2018.09.24 |
[Node.js] socket.io 와 Express 모듈을 이용한 간단 채팅 서버 구현 (0) | 2018.09.13 |
Express (Node.js를 위한 빠르고 간결한 개방 웹 프레임워크) (0) | 2018.09.12 |
CentOS6 node.js 서버 환경 구축 (0) | 2017.08.11 |